LoRa port for TARPN node: Difference between revisions
No edit summary |
No edit summary |
||
Line 189: | Line 189: | ||
# sudo mkdir /var/log/lora | # sudo mkdir /var/log/lora | ||
# sudo touch /var/log/lora/lora.log | # sudo touch /var/log/lora/lora.log | ||
# Replace KissHelper.py with this contents (this extends it to handle all KISS packets not just APRS, and makes it a little more tolerant of unexpected failures rather than just stopping the process at the first weird packet): | # Replace KissHelper.py with this contents (this extends it to handle all KISS packets not just APRS, and makes it a little more tolerant of unexpected failures rather than just stopping the process at the first weird packet, and segments too-large packets): | ||
<nowiki> | <nowiki> | ||
#!/usr/bin/python3 | #!/usr/bin/python3 |
Revision as of 02:17, 27 August 2023
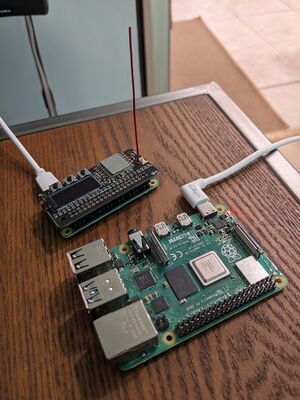
KV4P has been experimenting with adding LoRa ports to his TARPN node. LoRa is very desirable for TARPN because LoRa transceivers are very inexpensive yet have good range, are very small and low-power, and reduce the overall setup cost of a new link.
Materials:
- Raspberry Pi Zero 2 W (~$15 when in stock), WITH gpio header. You can use any Rapsberry Pi model for this, but this is what I used.
- Adafruit LoRa Radio Bonnet with OLED - RFM95W @ 915MHz - RadioFruit ($32.50)
- 78mm of 16AWG magnet wire (33cm band 1/4 wave antenna), soldered to the radio bonnet antenna center conductor via (right next to the connector we won't use) (~$12 for a spool)
This assumes you already have a TARPN node. With this experimental guide, you'll be building a LoRa radio that shows up on your network as a TCP-based KISS TNC, which we'll wrap in a virtual serial port so TARPN ports 11 or 12 can be configured to access it like a normal TNC.
I'm going to assume you already have the Raspberry Pi configured with Raspberry Pi OS, and connected to your local network (the same network as your TARPN node). You'll want to assign a static IP to it (rather than DHCP), so you can ensure your TARPN node will be able to find it on the network after restarts.
Get LoRa bonnet working
You can read more about these steps on this Adafruit page.
- sudo apt install python3-pip
- sudo pip3 install --upgrade setuptools
- sudo pip3 install --upgrade adafruit-python-shell
- wget https://github.com/adafruit/Adafruit_CircuitPython_framebuf/raw/main/examples/font5x8.bin
- wget https://raw.githubusercontent.com/adafruit/Raspberry-Pi-Installer-Scripts/master/raspi-blinka.py
- sudo python3 raspi-blinka.py (this will require reboot at the end)
- sudo pip3 install adafruit-circuitpython-ssd1306
- sudo pip3 install adafruit-circuitpython-framebuf
- sudo pip3 install adafruit-circuitpython-rfm9x
- create this test script rfm9x_check.py, which you should run ("python3 rfm9x_check.py") to prove your bonnet is properly connected and working:
# SPDX-FileCopyrightText: 2018 Brent Rubell for Adafruit Industries # # SPDX-License-Identifier: MIT """ Wiring Check, Pi Radio w/RFM9x Learn Guide: https://learn.adafruit.com/lora-and-lorawan-for-raspberry-pi Author: Brent Rubell for Adafruit Industries """ import time import busio from digitalio import DigitalInOut, Direction, Pull import board # Import the SSD1306 module. import adafruit_ssd1306 # Import the RFM9x radio module. import adafruit_rfm9x # Button A btnA = DigitalInOut(board.D5) btnA.direction = Direction.INPUT btnA.pull = Pull.UP # Button B btnB = DigitalInOut(board.D6) btnB.direction = Direction.INPUT btnB.pull = Pull.UP # Button C btnC = DigitalInOut(board.D12) btnC.direction = Direction.INPUT btnC.pull = Pull.UP # Create the I2C interface. i2c = busio.I2C(board.SCL, board.SDA) # 128x32 OLED Display reset_pin = DigitalInOut(board.D4) display = adafruit_ssd1306.SSD1306_I2C(128, 32, i2c, reset=reset_pin) # Clear the display. display.fill(0) display.show() width = display.width height = display.height # Configure RFM9x LoRa Radio CS = DigitalInOut(board.CE1) RESET = DigitalInOut(board.D25) spi = busio.SPI(board.SCK, MOSI=board.MOSI, MISO=board.MISO) while True: # Clear the image display.fill(0) # Attempt to set up the RFM9x Module try: rfm9x = adafruit_rfm9x.RFM9x(spi, CS, RESET, 915.0) display.text('RFM9x: Detected', 0, 0, 1) except RuntimeError as error: # Thrown on version mismatch display.text('RFM9x: ERROR', 0, 0, 1) print('RFM9x Error: ', error) # Check buttons if not btnA.value: # Button A Pressed display.text('Ada', width-85, height-7, 1) display.show() time.sleep(0.1) if not btnB.value: # Button B Pressed display.text('Fruit', width-75, height-7, 1) display.show() time.sleep(0.1) if not btnC.value: # Button C Pressed display.text('Radio', width-65, height-7, 1) display.show() time.sleep(0.1) display.show() time.sleep(0.1)
Turn it into a TCP KISS TNC
See the github project for more info.
- sudo apt install git aprx python3-rpi.gpio python3-spidev python3-pil python3-smbus
- git clone https://github.com/IZ7BOJ/RPi-LoRa-KISS-TNC-2ndgen.git
- cd RPi-LoRa-KISS-TNC-2ndgen
- git clone https://github.com/mayeranalytics/pySX127x.git
- sudo mv board_config.py pySX127x/SX127x/board_config.py
- sudo mv LoRa.py pySX127x/SX127x//LoRa.py
- Replace config.py with these contents. You can/should customize the frequency to anything in the 33cm band (but at least 500kHz from the band edge):
## Config file for RPi-LoRa-KISS-TNC 2nd generation ## Lora Module selection sx127x = True #if True, enables sx127x family, else sx126x ## Display enable and font disp_en = False font_size = 8 ## Log enable and path log_enable = True logpath='/var/log/lora/lora.log' #log filename. Give r/w permission! ## KISS Settings # Where to listen? # TCP_HOST can be "localhost", "0.0.0.0" or a specific interface address # TCP_PORT as configured in aprx.conf <interface> section TCP_HOST = "0.0.0.0" TCP_PORT = 10001 ## Hardware Settings # See datasheets for detailed pinout. # The default pin assignment refers to PCB designed by I8FUC. # The user can wire the module by his own and change pin assignment. # assign "-1" if the pin is not used # Settings valid for both SX126x and SX127x busId = 0 #SPI Bus ID. Must be enabled on raspberry (sudo raspi-config). Default is 0 csId = 1 #SPI Chip Select pin. Valid values are 0 or 1 irqPin = 22 #DIO0 of sx127x and DIO1 of sx126x, used for IRQ in rx # Settings valid only for SX126x. Default pin assignment refers to the PCB schematic /doc/LoRa_RPi_Companion_2022.pdf resetPin = 6 busyPin = 4 # If txen and rxen are disabled (=-1), then DIO2 will be set as RF Switch control txenPin = 0 #In Ebyte modules, it's used for switching on the tx pa. rxenPin = 1 #In Ebyte modules, it's used for switching on the rx lna # If Lora module has a TCXO, the following parameter must be True # If True, the DIO3 line will be set as control voltage of the TCXO tcxo=False ## LoRa Settings valid for both SX127x and SX126x modules frequency = 910300000 #frequency in Hz preamble = 8 #valid preable length is 8/16/24/32 spreadingFactor = 7 #valid spreading factor is between 7 and 12 bandwidth = 500000 #possible BW values: 7800, 10400,15600, 20800, 31250, 41700, 62500, 125000, 250000, 500000 codingrate = 5 #valid code rate denominator is between 5 and 8 appendSignalReport = False #append signal report when packets are forwarded to aprs server outputPower = 17 #maximum TX power is 22(22dBm) for SX126x, and 17 (17dBm) for SX127x . Higher values will be forced to max allowed! TX_OE_Style = False #if True, tx RF packets are in OE Style, otherwise in standard AX25 #sync_word = 0x1424 #sync word is x4y4. Es: 0x12 of 1st gen LoRa chip --> 0x1424 of 2nd gen LoRa chip sync_word = 0x12 crc = True #defines if CRC is calculated and transmitted in the header. Note that modem works in explicit mode #LoRa Settings valid only for SX126x RX_GAIN_POWER_SAVING = False #If false, receiver is set in boosted gain mode (needs more power)
The default settings above are for maximum throughput shorter-range links. If you want to go farther (at much slower speeds), try decreasing the bandwidth to 62500 and increasing the spreadingFactor to 8 (this will concentrate more power in a narrower signal, and slightly increase the encoding spread which takes longer to transmit the signal). These are the 2 most important values (bandwidth and spreadingFactor) in terms of throughput and range.
Note that if you're using a ham band other than 33cm you may need to use lower bandwidth settings to adhere to FCC requirements for spread spectrum transmissions (<100kHz bandwidth below 33cm). You can use the full 500kHz 33cm and above.
This github project needs more modification to work with TARPN, since it was originally written only for APRS packets and will break with any other kind. It also assumed ~400MHz, whereas the Adafruit bonnet is 33cm (902-928MHz). Here is how to update it (TODO these should be in a forked github project to remove these steps):
- nano pySX127x/SX127x/board_config.py (Change low_band = True, to False for 33cm support)
- nano pySX127x/SX127x/LoRa.py (Search for “43” and change multiple occurences to 915MHz)
- nano LoraAprsKissTnc_sx127x.py (Ditto, there's only 1 occurence in this one)
- sudo mkdir /var/log/lora
- sudo touch /var/log/lora/lora.log
- Replace KissHelper.py with this contents (this extends it to handle all KISS packets not just APRS, and makes it a little more tolerant of unexpected failures rather than just stopping the process at the first weird packet, and segments too-large packets):
#!/usr/bin/python3 # This program is free software: you can redistribute it and/or modify # it under the terms of the GNU General Public License as published by # the Free Software Foundation, either version 3 of the License, or # (at your option) any later version. # # This program is distributed in the hope that it will be useful, # but WITHOUT ANY WARRANTY; without even the implied warranty of # MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the # GNU General Public License for more details. # # You should have received a copy of the GNU General Public License # along with this program. If not, see <http://www.gnu.org/licenses/>. # Inspired by: # * Python script to decode AX.25 from KISS frames over a serial TNC # https://gist.github.com/mumrah/8fe7597edde50855211e27192cce9f88 # # * Sending a raw AX.25 frame with Python # https://thomask.sdf.org/blog/2018/12/15/sending-raw-ax25-python.html # # KISS-TNC for LoRa radio modem # https://github.com/IZ7BOJ/RPi-LoRa-KISS-TNC import struct import datetime import config KISS_FEND = 0xC0 # Frame start/end marker KISS_FESC = 0xDB # Escape character KISS_TFEND = 0xDC # If after an escape, means there was an 0xC0 in the source message KISS_TFESC = 0xDD # If after an escape, means there was an 0xDB in the source message def logf(message): timestamp = datetime.datetime.now().strftime('%Y/%m/%d %H:%M:%S - ') if config.log_enable: fileLog = open(config.logpath,"a") fileLog.write(timestamp + message+"\n") fileLog.close() print(timestamp + message) MAX_SEGMENT_LENGTH = 200 # for segmented packets segments = [] def encode_kiss_AX25(frame,signalreport): #from Lora to Kiss, Standard AX25 global segments if len(frame) > MAX_SEGMENT_LENGTH + 1: logf("Warning: Discarded very long frame, even longer than a segmented frame. Likely corrupted.") return # If this is a data segment, process it as such. if len(frame) >= MAX_SEGMENT_LENGTH + 1 or len(segments) > 0: if frame[0:1] == b'0': if len(segments) == 0: logf("Start of segmented data, caching to recombine.") else: logf("Continuation of segmented data, adding to cache.") segments.append(frame[1:MAX_SEGMENT_LENGTH + 1]) return elif frame[0:1] == b'1': if len(segments) == 0: logf("Warning, corrupt segmentation, received end segment with no prior segments. Discarding.") segments = [] return else: logf("End of segmented data, adding to cache and returning complete packet via KISS.") segments.append(frame[1:]) frame = bytearray() for segment in segments: for b in segment: frame.append(b) segments = [] packet_escaped = [] for x in frame: if x == KISS_FEND: packet_escaped += [KISS_FESC, KISS_TFEND] elif x == KISS_FESC: packet_escaped += [KISS_FESC, KISS_TFESC] else: packet_escaped += [x] kiss_cmd = 0x00 # Two nybbles combined - TNC 0, command 0 (send data) kiss_frame = [KISS_FEND, kiss_cmd] + packet_escaped + [KISS_FEND] try: output = bytearray(kiss_frame) except ValueError: logf("Invalid value in frame.") return None return output def decode_kiss_AX25(frame): #from kiss to LoRA, Standard AX25 result = b"" if frame[0] != 0xC0 or frame[len(frame) - 1] != 0xC0: logf("Kiss Header not found, abort decoding of Frame: "+repr(frame)) return None frame=frame[2:len(frame) - 1] #cut kiss delimitator 0xc0 and command 0x00 return frame class SerialParser(): '''Simple parser for KISS frames. It handles multiple frames in one packet and calls the callback function on each frame''' STATE_IDLE = 0 STATE_FEND = 1 STATE_DATA = 2 KISS_FEND = KISS_FEND def __init__(self, frame_cb=None): self.frame_cb = frame_cb self.reset() def reset(self): self.state = self.STATE_IDLE self.cur_frame = bytearray() def parse(self, data): try: for c in data: if self.state == self.STATE_IDLE: if c == self.KISS_FEND: self.cur_frame.append(c) self.state = self.STATE_FEND elif self.state == self.STATE_FEND: if c == self.KISS_FEND: self.reset() else: self.cur_frame.append(c) self.state = self.STATE_DATA elif self.state == self.STATE_DATA: self.cur_frame.append(c) if c == self.KISS_FEND: # frame complete if self.frame_cb: self.frame_cb(self.cur_frame) self.reset() except Exception as e: logf("Exception in SerialParser.parse(), callback NOT called.") logf(str(e))
Start/test your TCP-based KISS TNC with this command (you should see it print out the config values and say it's listening for connections, with no errors listed):
- sudo python3 Start_lora-tnc.py
This is what you should see:
######################## #LORA KISS TNC STARTING# ######################## #Lora parameters: frequency= 910300000 preamble= 32 spreadingFactor= 7 bandwidth= 500000 codingrate= 5 APPEND_SIGNAL_REPORT= False outputPower= 17 TX_OE_Style= False sync_word= 0x12 crc= True ######################## 2023/08/15 00:27:18 - KISS-Server: Started. Listening on IP 0.0.0.0 Port: 10001 2023/08/15 00:27:18 - LoRa radio initialized. Waiting for LoRa Spots...
When you run the TNC for long-term use, you'll want to run it with "nohup sudo python3 Start_lora-tnc.py &" which will disassociate it with your linux account and run it in the background until manually terminated. In the future, we should wrap this in a systemd service, or you can start it in crontab.
TARPN configuration
TARPN only supports serial TNCs, so first we need to "fake" a serial TNC that actually connects to our TCP-bsaed LoRa KISS TNC using the "socat" command.
- sudo apt install socat
- sudo nano lora-port-up.sh
- Use these contents but replace the IP address to your LoRa KISS TNC's address:
#!/bin/bash printf "Bringing up LoRa port as /dev/ttyS0\n" while true do sudo socat pty,link=/dev/ttyS0,b115200,raw,echo=0,mode=777 tcp:192.168.1.90:10001,forever,interval=10 printf "LoRa port /dev/ttyS0 disconnected, waiting 1 second and bringing it up again.\n" sleep 1 done
- Start the fake serial device with "./lora-port-up.sh &" and it will show up as /dev/ttyS0 like a serial TNC would be. (You might want to do this in crontab or something, so it always runs on node restart.)
Then you can edit your TARPN node.ini to configure port 11 as follows:
usb-port11:ENABLE portdev11:/dev/ttyS0 speed11:115200 txdelay11:1 frack11:2000 kissoptions11:disable neighbor11:KV4P-3
Of course, assign "neighbor11" to the callsign/ssid of the node you plan to connect to via LoRa on your new port 11.
Performance
With the above config.py settings, a throughput test on my own TARPN node with a "bench setup" (2 LoRa transceivers right next to each other), I achieved 135bytes/sec. That's about as good as a very solid 9600 baud link with a NinoTNC and high quality mobile radios.
It can probably be made to go much faster with additional optimization. It's unclear if the KISS service running in Python represents a cap on the throughput, or if it's just the settings.
Although the distance of this link hasn't been tested yet, the LoRa module claims to work between 4km and 40km (from city to perfectly flat ideal conditions). 33cm has the nice property of easily going through windows and walls, so it should be especially good for suburban links, or between nodes with a fairly clear shot.